ソース
import java.awt.*;
import java.awt.event.*;
public class CtoF extends Frame {
Button but = new Button("変換");
Label lab = new Label("温度を入力して下さい");
TextField txt = new TextField();
Checkbox cb0 = new Checkbox("華氏→摂氏");
Checkbox cb1 = new Checkbox("摂氏→華氏");
CheckboxGroup cbG = new CheckboxGroup();
public CtoF() {
setLayout(null);
add(txt); txt.setBounds(10, 40, 90, 30);
add(but); but.setBounds(110, 40, 80, 30);
add(lab); lab.setBounds(10, 90, 180, 20);
cb0.setCheckboxGroup(cbG);
cb1.setCheckboxGroup(cbG);
cb0.setState(true);
add(cb0); cb0.setBounds(10, 105, 100, 15);
add(cb1); cb1.setBounds(10, 120, 100, 15);
but.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
String str;
int i;
try{
double f = Double.parseDouble(txt.getText());
if(cb0.getState() == true){
str = Double.toString((f-32.0)*5.0/9.0);
i = str.indexOf('.');
//System.out.println(str);
lab.setText(f+"F = "
+str.concat("000").substring(0,i+3)+"℃");
}else{
str = Double.toString((f*9.0/5.0)+32);
i = str.indexOf('.');
//System.out.println(str);
lab.setText(f+"。?= "
+str.concat("000").substring(0,i+3)+"F");
}
}catch(Exception e){
lab.setText("数値のみを入力して下さい(半角)");
}
txt.requestFocus();
txt.setText("");
}
});
}
public static void main(String[] args) {
Frame win = new CtoF();
win.setSize(200, 150); win.setVisible(true);
win.setTitle("摂氏華氏変換");
win.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent evt) {
System.exit(0);
}
});
}
}
実行結果
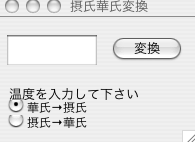
考察
奇数偶数判定プログラムと似たような処理。こっちが、難しい。
5.「電卓」プログラム。中身は自分の思うように。
ソース
import java.awt.*;
import java.awt.event.*;
import java.applet.Applet;
public class Keisannki extends Applet{
long temp = 0;
long temp2 = 0;
String x = "=";
boolean input = false;
boolean input2 = false;
int count = 0;
TextField t0 = new TextField("0");
Label l0 = new Label("");
Button b0 = new Button("0");
Button b1 = new Button("1");
Button b2 = new Button("2");
Button b3 = new Button("3");
Button b4 = new Button("4");
Button b5 = new Button("5");
Button b6 = new Button("6");
Button b7 = new Button("7");
Button b8 = new Button("8");
Button b9 = new Button("9");
Button bC = new Button("C");
Button bAC= new Button("AC");
Button ba = new Button("+");
Button bb = new Button("-");
Button bc = new Button("×");
Button bd = new Button("÷");
Button be = new Button("=");
class Buttonevent implements ActionListener {/*数字ボタンイベン
ト用リスナ*/
public void actionPerformed(ActionEvent ae){
if(input == true){/*lv1 符号入力有り(符号入力あ
り、初期化必要)*/
if(input2 == true){/*lv2 符号入力による
初期化判定(初期化済み)*/
if(count == 0){/*lv3 既入力判定
(初入力)*/
if(ae.getActionCommand()
!= "0"){/*lv4 0入力判定(0入力でない)*/
t0.setText(ae.getActionCommand());
count++;
}else{/*lv4 0入力判定(0
入力)*/
t0.setText(ae.getActionCommand());
}
}else{/*lv3 既入力判定(既入力)
*/
t0.setText(t0.getText()
+ ae.getActionCommand());
count++;
}
}else{/*lv2 符号入力による初期化判定(初
期化前)*/
if(ae.getActionCommand() !=
"0"){/*lv3 0入力判定(0入力でない)*/
t0.setText(ae.getActionCommand());
count++;
}else{/*lv3 0入力判定(0入力)*/
t0.setText(ae.getActionCommand());
}
input2 = true;
}
}else{/*lv1 符号入力判定(符号入力無し、初期化不
必要)*/
if(count == 0){/*lv2 既入力判定(初入力)
*/
if(ae.getActionCommand() !=
"0"){/*lv3 0入力判定(0入力でない)*/
t0.setText(ae.getActionCommand());
count++;
}else{/*lv3 0入力判定(0入力)*/
t0.setText("0");
}
}else{/*lv2 既入力判定(既入力)*/
t0.setText(t0.getText() +
ae.getActionCommand());
count++;
}
}
}
}
class Equalevent implements ActionListener {/*演算イベント用リス
ナ*/
public void actionPerformed(ActionEvent ae){
long temp3;
try{/*エラー発生箇所*/
if(x != "="){/*lv1 最低1回の符号入力の有
無判定(有り)*/
if(input != true){/*lv2 符号変更
判定(変更無し)*/
temp =
Long.parseLong(t0.getText());
}else{/*lv2 符号変更判定(変更有
り)*/
temp2 =
Long.parseLong(t0.getText());
}
if(x == "+"){/*lv2 演算条件群*/
t0.setText(Long.toString(temp+temp2));
}else{
if(x == "-"){
t0.setText(Long.toString(temp-temp2));
}else{
if(x == "*"){
t0.setText(Long.toString(temp*temp2));
}else{
if(x == "/"){
t0.setText(Long.toString(temp/temp2));
}}}}
if(Long.parseLong(t0.getText())
== 0){/*結果が0の時を例外として処理*/
count = 0;
}
input = false;
input2 = false;
}
}catch(Exception ex){/*エラー処理*/
l0.setText("E");
t0.setText("0");
count = 0;
temp = 0;
temp2 = 0;
x = "=";
input = false;
input2 = false;
}
}
}
class Xevent implements ActionListener {/*符号変更イベント用リス
ナ*/
public void actionPerformed(ActionEvent ae){
try{/*エラー発生箇所*/
temp =
Long.parseLong(t0.getText());
x = ae.getActionCommand();
input = true;
count = 0;
l0.setText(ae.getActionCommand());
}catch(Exception ex){/*エラー処理*/
l0.setText("E");
t0.setText("0");
count = 0;
temp = 0;
temp2 = 0;
x = "=";
input = false;
input2 = false;
}
}
}
public void init() {/*コンストラクタ(レイアウト、イベント設定)
*/
setLayout(null);/*レイアウトマネージャのカット*/
add(t0); t0.setBounds(50, 40, 150, 20); /*以下、レイアウ
トの決め打ち*/
t0.setEditable(false);
add(l0); l0.setBounds(20, 40, 30, 20);
add(b0); b0.setBounds(10, 240, 40, 30);
add(b1); b1.setBounds(10, 200, 40, 30);
add(b4); b4.setBounds(10, 160, 40, 30);
add(b7); b7.setBounds(10, 120, 40, 30);
add(b2); b2.setBounds(60, 200, 40, 30);
add(b5); b5.setBounds(60, 160, 40, 30);
add(b8); b8.setBounds(60, 120, 40, 30);
add(b3); b3.setBounds(110, 200, 40, 30);
add(b6); b6.setBounds(110, 160, 40, 30);
add(b9); b9.setBounds(110, 120, 40, 30);
add(be); be.setBounds(60, 240, 90, 30);
add(bC); bC.setBounds(110, 80, 40, 30);
add(bAC); bAC.setBounds(10, 80,90 ,30);
add(ba); ba.setBounds(170, 120, 40, 30);
add(bb); bb.setBounds(170, 160, 40, 30);
add(bc); bc.setBounds(170, 200, 40, 30);
add(bd); bd.setBounds(170, 240, 40, 30);
b0.addActionListener(new Buttonevent());/*以下、イベント
のリスナを登録*/
b1.addActionListener(new Buttonevent());
b2.addActionListener(new Buttonevent());
b3.addActionListener(new Buttonevent());
b4.addActionListener(new Buttonevent());
b5.addActionListener(new Buttonevent());
b6.addActionListener(new Buttonevent());
b7.addActionListener(new Buttonevent());
b8.addActionListener(new Buttonevent());
b9.addActionListener(new Buttonevent());
bC.addActionListener(new ActionListener(){/*Clearボタン
(無名クラスリスナ)*/
public void actionPerformed(ActionEvent ae){
t0.setText("0");
count = 0;
}
});
bAC.addActionListener(new ActionListener(){/*AllClearボ
タン(無名クラスリスナ)*/
public void actionPerformed(ActionEvent ae){
t0.setText("0");
l0.setText("");
count = 0;
temp = 0;
temp2 = 0;
x = "=";
input = false;
input2 = false;
}
});
be.addActionListener(new Equalevent());
ba.addActionListener(new Xevent());
bb.addActionListener(new Xevent());
bc.addActionListener(new Xevent());
bd.addActionListener(new Xevent());
}
public static void main(String[] args) {
Frame win = new GUIaa();
win.setSize(300, 410); win.setVisible(true);
win.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent evt) {
System.exit(0);
}
});
}
}
|