1.偶数奇数判定プログラム(GUIaa)をタイプし、その動作を考察せよ。
2.例外処理について、考察せよ。
3.上述のサンプルプログラムに出てきたGUI部品を、全て使ったプログラムを作成せよ。
4.摂氏から華氏、華氏から摂氏への温度換算ができるプログラムを作成せよ。
5.「電卓」プログラム。中身は自分の思うように。
1.偶数奇数判定プログラム(GUIaa)をタイプし、その動作を考察せよ。
1-1.プログラム
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
|
import java.awt.*;
import java.awt.event.*;
public class GUIaa extends Frame {
Button b0 = new Button("Even/Odd?"); //ボタンの生成
Label x0 = new Label("Type a number and press..."); //ラベルの生成
TextField t0 = new TextField(); //テキストフィールドの生成
public GUIaa() {
setLayout(null); //部品の自動配置機能をオフにする
add(t0); t0.setBounds(10, 40, 90, 30); //(左上隅のx/y座標、幅、高さ)
add(b0); b0.setBounds(110, 40, 80, 30);
add(x0); x0.setBounds(10, 80, 180, 30);
b0.addActionListener(new ActionListener() { //ボタンを押した後の動作
public void actionPerformed(ActionEvent evt) {
int i = (new Integer(t0.getText())).intValue();
t0.setText("");
if(i % 2 == 0) {
x0.setText(i + " is Even");
} else {
x0.setText(i + " is Odd");
}
}
});
}
public static void main(String[] args) {
Frame win = new GUIaa();
win.setSize(200, 150); win.setVisible(true); //ウィンドウの大きさ
win.addWindowListener(new WindowAdapter() { //ディスプレイに表示
public void windowClosing(WindowEvent evt) {
//ウィンドウを閉じた後の動作
System.exit(0); //プログラムを終了
}
});
}
}
|
1-2.実行結果
1-3.考察
入力した値が偶数か奇数かを判断するプログラムである。
4行目〜6行目のButton、Label、TextFirldの括弧内でボタンに表示する文字を決める。
9行目のsetLayout(null)はボタンやラベルテキストフィールド等の配置を自動で決めずに、こちらで全て設定するということを表している。
14行目ActionEventはボタンを押したときに生成される。
15行目int i = (new Integer(t0.getText())).intValue();
はt0.getText()でt0の文字(入力された値)を読み込みIntegerで生成後のオブジェクトを不変なものとする。
29行目windowClosingはユーザーがウィンドウのクローズを要求する時このメソッドが呼び出される。
またWindowEventはユーザーがウィンドウを活動化したとき、閉じたとき、非活動化したとき、アイコン化解除したとき、アイコン化したとき、開いたとき、終了したときに生成される。
2.例外処理について、考察せよ。
2-1.プログラム
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
|
import java.awt.*;
import java.awt.event.*;
public class ColorRGBa extends Frame { // Frameクラスの継承
Button b0 = new Button("Display"); // ボタンの中身
Label[] la = new Label[]{new Label ("Red"), // 配列
new Label ("Green"),
new Label ("Blue")};
TextField[] ta = new TextField[]{new TextField(), // 配列
new TextField(),
new TextField()};
Label x0 = new Label("Input RGB values [0..255]");
public ColorRGBa() {
setLayout(null); // 部品の自動配置機能をオフにする
// LabelとTextFieldをy座標40の間隔で貼付ける
for(int i = 0; i < la.length; i++) {
add(la[i]); la[i].setBounds(10, 40 + i*40, 60, 30);
add(ta[i]); ta[i].setBounds(80, 40 + i*40, 60, 30);
}
add(b0); b0.setBounds(10, 160, 60, 30); // ボタンの貼付け
add(x0); x0.setBounds(10, 200, 180, 30); // 表示欄の貼付け
b0.addActionListener(new ActionListener() { // ボタンを押した後の動作
public void actionPerformed(ActionEvent evt) {
try {
x0.setBackground(new Color( // 背景色の設定
(new Integer(ta[0].getText())).intValue(),
(new Integer(ta[1].getText())).intValue(),
(new Integer(ta[2].getText())).intValue()));
// エラーメッセージの表示
} catch(Exception ex) { x0.setText(ex.toString()); }
}
});
}
public static void main(String[] args) {
Frame win = new ColorRGBa();
// 前述のオブジェクトを、変数winへ生成・初期化
win.setSize(200, 250); // ウィンドウの大きさ
win.setVisible(true); // ディスプレイへの表示
win.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent evt) {
//ウィンドウを閉じた後の動作
System.exit(0); // 現在のプログラムを終了する
}
});
}
}
|
2-2.実行結果
2-3.考察
try {
…例外が発生する可能性のある処理…
} catch(Exception ex) {
…例外時の処理…
Javaでは、あらゆる種類の「想定外の事柄」(エラー等)はすべて例外(exception)と呼ばれる動作の発生によって通知される。
例外が発生したときそれを処理するには、このようなtry文を用いる。
try {
x0.setBackground(new Color(
(newInteger(ta[0].getText())).intValue(),
(newInteger(ta[1].getText())).intValue(),
(newInteger(ta[2].getText())).intValue()));
} catch(Exception ex) { x0.setText(ex.toString()); }
}
「try{}文」で囲まれているため、例外が起こると文末の「catch(Exceptionex)」以下の処理が行われる。
ここでは、入力された3つの値(0~255)によってラベルの背景カラーが変わる。
プログラムで、処理できない値が入力されると 背景カラーが変わる代わりに、エラー文を表示する。
3.上述のサンプルプログラムに出てきたGUI部品を、全て使ったプログラムを作成せよ。
3-1.プログラム
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
|
import java.awt.*;
import java.awt.event.*;
public class report5_3 extends Frame {
int i; char c;
Label x0 = new Label("Name");
TextField t0 = new TextField();
Checkbox c0 = new Checkbox("Student?");
List l1 = new List();
TextArea t1 = new TextArea("Free Write");
Choice c1 = new Choice();
Choice c2 = new Choice();
Button b0 = new Button("ENTER");
TextField t2 = new TextField();
public report5_3() {
setLayout(null); // 部品の自動配置機能をオフにする
add(x0); x0.setBounds(20, 50, 60, 30); // 表示欄の貼付け
add(t0); t0.setBounds(70, 50, 300, 30); // 入力欄の大きさ
add(c0); c0.setBounds(170, 100, 80, 30); // Checkboxの貼付け
add(l1); l1.setBounds(30, 100, 100, 210); // Listの大きさ
add(t1); t1.setBounds(170, 210, 200, 100); // 入力欄の大きさ
// Listの中身
for(i=15; i<31; i++)
l1.add("Age"+i);
add(c1); c1.setBounds(170, 125, 100, 50); // Choice1の貼付け
// Choice1の中身
c1.add("male"); c1.add("female");
add(c2); c2.setBounds(170, 160, 150, 50); // Choice2の貼付け
// Choice2の中身
c2.add("Hokkaido"); c2.add("Touhoku"); c2.add("Kantou");
c2.add("Tyubu"); c2.add("Kansai"); c2.add("Kinki");
c2.add("Sikoku,Tyugoku"); c2.add("Kyusyu");
add(b0); b0.setBounds(150, 320, 80, 30); // ボタンの貼付け
// ボタンの中身
b0.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
t2.setText("YATTAAAA!!!");
}
});
add(t2); t2.setBounds(145, 350, 90, 30); // テキスト
}
public static void main(String[] args) {
Frame win = new report5_3(); // 前述のオブジェクトを、変数winへ生成・初期化
win.setSize(400, 400); // ウィンドウの大きさ
win.setVisible(true); // ディスプレイへの表示
}
}
|
3-2.実行結果
3-3.考察
このjavaは「ENTER」のボタンを押すと「YATTAAAA!!!」と表示されるプログラミングである。
名前・年齢・学生・性別・地域など様々な設定を入力できるが、表示される言葉に一切関係はない。
6〜14行目は使用するGUIを設定している。
18〜22は表示欄、入力欄、チェックボックス、リストの位置をそれぞれ設定している。
25,26行目はfor文を使ってAge15〜Age30までを出力している。
45〜47はボタンの設定で、ボタンが押されたら文字を表示するようにしている。
4.摂氏から華氏、華氏から摂氏への温度換算ができるプログラムを作成せよ。
4-1.プログラム
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62
|
import java.awt.*;
import java.awt.event.*;
public class report5_4 extends Frame{
Label x0 = new Label("temperature");
Button b0 = new Button("K -> C");
Button b1 = new Button("C -> K");
Button b2 = new Button("clear");
Label x1 = new Label("choice");
Label x3 = new Label("temp");
TextField t0 = new TextField();
public report5_4() {
setLayout(null);
add(x0); x0.setBounds(30, 40, 80, 20);
add(b0); b0.setBounds(140, 50, 80, 20);
add(b1); b1.setBounds(140, 70, 80, 20);
add(b2); b2.setBounds(148, 100, 65, 20);
add(x1); x1.setBounds(155, 30, 50, 20);
add(x3); x3.setBounds(240, 60, 48, 20);
add(t0); t0.setBounds(40, 60, 50, 20);
b0.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
try {
int i = (new
Integer(t0.getText())).intValue();
x3.setText("" + i*1.8+32);
}catch(Exception ex) {
x3.setText("error");
}
}
});
b1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
try {
int i = (new
Integer(t0.getText())).intValue();
x3.setText("" + (i-32)/1.8);
} catch (Exception ex) {
x3.setText("error");
}
}
});
b2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
t0.setText(""); x3.setText("temp");
}
});
}
public static void main(String[] args) {
Frame win = new report5_4();
win.setSize(350, 150);
win.setVisible(true);
win.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent evt) {
System.exit(0);
}
});
}
}
|
4-2.実行結果
4-3.考察
摂氏から華氏への変換は「摂氏*1.8+32」である。
また華氏から摂氏への変換は逆に「(華氏-32)/1.8」である。
数字以外が入力されるとtry文によって「error」が表示される。
残りの部分は上のGUIと同じような仕組みである。
5.「電卓」プログラム。中身は自分の思うように。
5-1.プログラム
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92
|
import java.awt.*;
import java.awt.event.*;
public class report5_5 extends Frame {
Button b0 = new Button("+");
Button b1 = new Button("*");
Button b2 = new Button("-");
Button b3 = new Button("/");
TextField t0 = new TextField();
TextField t1 = new TextField();
TextField t2 = new TextField();
Label l0 = new Label("calculation");
public report5_5() {
setLayout(null);
add(t0); t0.setBounds(50, 40, 90, 30);
add(t1); t1.setBounds(50, 80, 90, 30);
add(t2); t2.setBounds(50, 140, 90, 30);
add(b0); b0.setBounds(10, 40, 35, 35);
add(b1); b1.setBounds(10, 80, 35, 35);
add(b2); b2.setBounds(150, 40, 35, 35);
add(b3); b3.setBounds(150, 80, 35, 35);
add(l0); l0.setBounds(60, 120, 110,40);
b0.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
try{
double a = (new Double(t0.getText())).doubleValue();
double b = (new Double(t1.getText())).doubleValue();
double c = a + b;
t2.setText("" + c);
l0.setText("addition");
}catch(Exception ex){
l0.setText("error");
t2.setText("");
}
}
});
b1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
try{
double a = (new Double(t0.getText())).doubleValue();
double b = (new Double(t1.getText())).doubleValue();
double c = a * b;
t2.setText("" + c);
l0.setText("subtraction");
}catch(Exception ex){
l0.setText("error");
t2.setText("");
}
}
});
b2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
try{
double a = (new Double(t0.getText())).doubleValue();
double b = (new Double(t1.getText())).doubleValue();
double c = a - b;
t2.setText("" + c);
l0.setText("multiplication");
}catch(Exception ex){
l0.setText("error");
t2.setText("");
}
}
});
b3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
try{
double a = (new Double(t0.getText())).doubleValue();
double b = (new Double(t1.getText())).doubleValue();
double c = a / b;
t2.setText("" + c);
l0.setText("division");
}catch(Exception ex){
l0.setText("error");
t2.setText("");
}
}
});
}
public static void main(String[] args) {
Frame win = new report5_5();
win.setSize(200, 200); win.setVisible(true);
win.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent evt) {
System.exit(0);
}
});
}
}
|
5-2.実行結果
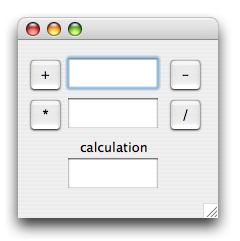
5-3.考察
上のテキストフィールドに演算される数値を入力し、真ん中のテキストフィールドに演算する数値を入力する。答えは一番下のテキストフィールドに表示される。
デザインを重視してみたかったが結局は使いづらくなりそうなのでどちらも良くなるようにしたつもりである。
数値以外を入力するとerrorを表示させるためtry文を用いている。
double型なので入力した数値や演算結果が8桁を越えるとオーバーフローを起こして正確な値が返ってこなくなることに注意。
|